On this tutorial, we’ll learn to leverage the Adala framework to construct a modular lively studying pipeline for medical symptom classification. We start by putting in and verifying Adala alongside required dependencies, then combine Google Gemini as a customized annotator to categorize signs into predefined medical domains. By means of a easy three-iteration lively studying loop, prioritizing crucial signs comparable to chest ache, we’ll see easy methods to choose, annotate, and visualize classification confidence, gaining sensible insights into mannequin habits and Adala’s extensible structure.
!pip set up -q git+https://github.com/HumanSignal/Adala.git
!pip record | grep adala
We set up the most recent Adala launch straight from its GitHub repository. On the similar time, the next pip record | grep adala command scans your setting’s bundle record for any entries containing “adala,” offering a fast affirmation that the library was put in efficiently.
import sys
import os
print("Python path:", sys.path)
print("Checking if adala is in put in packages...")
!discover /usr/native -name "*adala*" -type d | grep -v "__pycache__"
!git clone https://github.com/HumanSignal/Adala.git
!ls -la Adala
We print out your present Python module search paths after which search the /usr/native listing for any put in “adala” folders (excluding __pycache__) to confirm the bundle is on the market. Subsequent, it clones the Adala GitHub repository into your working listing and lists its contents so you may affirm that every one supply recordsdata have been fetched appropriately.
import sys
sys.path.append('/content material/Adala')
By appending the cloned Adala folder to sys.path, we’re telling Python to deal with /content material/Adala as an importable bundle listing. This ensures that subsequent import Adala… statements will load straight out of your native clone moderately than (or along with) any put in model.
!pip set up -q google-generativeai pandas matplotlib
import google.generativeai as genai
import pandas as pd
import json
import re
import numpy as np
import matplotlib.pyplot as plt
from getpass import getpass
We set up the Google Generative AI SDK alongside data-analysis and plotting libraries (pandas and matplotlib), then import key modules, genai for interacting with Gemini, pandas for tabular knowledge, json and re for parsing, numpy for numerical operations, matplotlib.pyplot for visualization, and getpass to immediate the person for his or her API key securely.
strive:
from Adala.adala.annotators.base import BaseAnnotator
from Adala.adala.methods.random_strategy import RandomStrategy
from Adala.adala.utils.custom_types import TextSample, LabeledSample
print("Efficiently imported Adala elements")
besides Exception as e:
print(f"Error importing: {e}")
print("Falling again to simplified implementation...")
This strive/besides block makes an attempt to load Adala’s core courses, BaseAnnotator, RandomStrategy, TextSample, and LabeledSample in order that we will leverage its built-in annotators and sampling methods. On success, it confirms that the Adala elements can be found; if any import fails, it catches the error, prints the exception message, and gracefully falls again to an easier implementation.
GEMINI_API_KEY = getpass("Enter your Gemini API Key: ")
genai.configure(api_key=GEMINI_API_KEY)
We securely immediate you to enter your Gemini API key with out echoing it to the pocket book. Then we configure the Google Generative AI shopper (genai) with that key to authenticate all subsequent calls.
CATEGORIES = ("Cardiovascular", "Respiratory", "Gastrointestinal", "Neurological")
class GeminiAnnotator:
def __init__(self, model_name="fashions/gemini-2.0-flash-lite", classes=None):
self.mannequin = genai.GenerativeModel(model_name=model_name,
generation_config={"temperature": 0.1})
self.classes = classes
def annotate(self, samples):
outcomes = ()
for pattern in samples:
immediate = f"""Classify this medical symptom into considered one of these classes:
{', '.be a part of(self.classes)}.
Return JSON format: {{"class": "selected_category",
"confidence": 0.XX, "clarification": "brief_reason"}}
SYMPTOM: {pattern.textual content}"""
strive:
response = self.mannequin.generate_content(immediate).textual content
json_match = re.search(r'({.*})', response, re.DOTALL)
end result = json.hundreds(json_match.group(1) if json_match else response)
labeled_sample = sort('LabeledSample', (), {
'textual content': pattern.textual content,
'labels': end result("class"),
'metadata': {
"confidence": end result("confidence"),
"clarification": end result("clarification")
}
})
besides Exception as e:
labeled_sample = sort('LabeledSample', (), {
'textual content': pattern.textual content,
'labels': "unknown",
'metadata': {"error": str(e)}
})
outcomes.append(labeled_sample)
return outcomes
We outline a listing of medical classes and implement a GeminiAnnotator class that wraps Google Gemini’s generative mannequin for symptom classification. In its annotate technique, it builds a JSON-returning immediate for every textual content pattern, parses the mannequin’s response right into a structured label, confidence rating, and clarification, and wraps these into light-weight LabeledSample objects, falling again to an “unknown” label if any errors happen.
sample_data = (
"Chest ache radiating to left arm throughout train",
"Persistent dry cough with occasional wheezing",
"Extreme headache with sensitivity to gentle",
"Abdomen cramps and nausea after consuming",
"Numbness in fingers of proper hand",
"Shortness of breath when climbing stairs"
)
text_samples = (sort('TextSample', (), {'textual content': textual content}) for textual content in sample_data)
annotator = GeminiAnnotator(classes=CATEGORIES)
labeled_samples = ()
We outline a listing of uncooked symptom strings and wrap every in a light-weight TextSample object to move them to the annotator. It then instantiates your GeminiAnnotator with the predefined class set and prepares an empty labeled_samples record to retailer the outcomes of the upcoming annotation iterations.
print("nRunning Energetic Studying Loop:")
for i in vary(3):
print(f"n--- Iteration {i+1} ---")
remaining = (s for s in text_samples if s not in (getattr(l, '_sample', l) for l in labeled_samples))
if not remaining:
break
scores = np.zeros(len(remaining))
for j, pattern in enumerate(remaining):
scores(j) = 0.1
if any(time period in pattern.textual content.decrease() for time period in ("chest", "coronary heart", "ache")):
scores(j) += 0.5
selected_idx = np.argmax(scores)
chosen = (remaining(selected_idx))
newly_labeled = annotator.annotate(chosen)
for pattern in newly_labeled:
pattern._sample = chosen(0)
labeled_samples.lengthen(newly_labeled)
newest = labeled_samples(-1)
print(f"Textual content: {newest.textual content}")
print(f"Class: {newest.labels}")
print(f"Confidence: {newest.metadata.get('confidence', 0)}")
print(f"Clarification: {newest.metadata.get('clarification', '')(:100)}...")
This lively‐studying loop runs for 3 iterations, every time filtering out already‐labeled samples and assigning a base rating of 0.1—boosted by 0.5 for key phrases like “chest,” “coronary heart,” or “ache”—to prioritize crucial signs. It then selects the best‐scoring pattern, invokes the GeminiAnnotator to generate a class, confidence, and clarification, and prints these particulars for overview.
classes = (s.labels for s in labeled_samples)
confidence = (s.metadata.get("confidence", 0) for s in labeled_samples)
plt.determine(figsize=(10, 5))
plt.bar(vary(len(classes)), confidence, shade="skyblue")
plt.xticks(vary(len(classes)), classes, rotation=45)
plt.title('Classification Confidence by Class')
plt.tight_layout()
plt.present()
Lastly, we extract the anticipated class labels and their confidence scores and use Matplotlib to plot a vertical bar chart, the place every bar’s peak displays the mannequin’s confidence in that class. The class names are rotated for readability, a title is added, and tight_layout() ensures the chart components are neatly organized earlier than show.
In conclusion, by combining Adala’s plug-and-play annotators and sampling methods with the generative energy of Google Gemini, we’ve constructed a streamlined workflow that iteratively improves annotation high quality on medical textual content. This tutorial walked you thru set up, setup, and a bespoke GeminiAnnotator, and demonstrated easy methods to implement priority-based sampling and confidence visualization. With this basis, you may simply swap in different fashions, broaden your class set, or combine extra superior lively studying methods to deal with bigger and extra advanced annotation duties.
Take a look at Colab Pocket book right here. All credit score for this analysis goes to the researchers of this venture. Additionally, be at liberty to observe us on Twitter and don’t neglect to hitch our 90k+ ML SubReddit.
Right here’s a quick overview of what we’re constructing at Marktechpost:
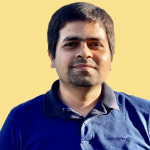
Asif Razzaq is the CEO of Marktechpost Media Inc.. As a visionary entrepreneur and engineer, Asif is dedicated to harnessing the potential of Synthetic Intelligence for social good. His most up-to-date endeavor is the launch of an Synthetic Intelligence Media Platform, Marktechpost, which stands out for its in-depth protection of machine studying and deep studying information that’s each technically sound and simply comprehensible by a large viewers. The platform boasts of over 2 million month-to-month views, illustrating its recognition amongst audiences.